Click For Python Variables Part 2
Variables Output Using Plus (+) And Comma (,)
We use print()
function to print the variable values. For example:
Code:
a = "Python"
print(a)
Output:
Python
If we have multiple variables.
Code:
a = "Python"
b = "Java"
c = "JavaScript"
print(a)
print(b)
print(c)
Output:
Python
Java
JavaScript
In this example, we use the multiple print function for output. Instead of using the multiple print function, we can use the single print function for output. We can use either the add (+) or comma (,) sign.
Using Add (+) Symbol
In the upper example, instead of using the multiple print function, we can use the single print function. For example:
Code:
a = "Python"
b = "Java"
c = "JavaScript"
print(a+b+c)
Output:
PythonJavaJavaScript
We get our result in one line. We didn’t get any space between our values. We can adjust it by providing space in our values.
Code:
a = "Python "
b = "Java "
c = "JavaScript"
print(a + b + c)
Output:
Python Java JavaScript
We simply provide space after each value we write.
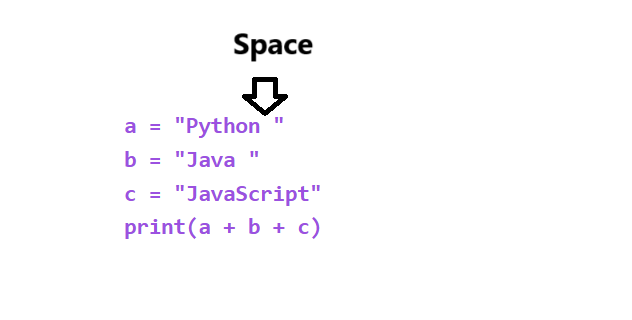
Using Comma (,) Symbol
Now what if we use a comma instead of an add symbol?
Code:
a = "Python"
b = "Java"
c = "JavaScript"
print(a, b, c)
Output:
Python Java JavaScript
We almost get the same result. Also, we get the space between values. This is the one difference between the add (+) and comma (,) sign but there is a major difference between both of them.
Difference Between Add (+) And Comma (,) While Printing
In upper example. It looks like we get the same result but it is not. When we use the add symbol, it means we are adding those values. And when we use the comma symbol, it means we are just printing those values. For example:
Example 1:
Code:
a = "Python"
b = 100
print(a, b)
Output:
Python 100
Example 2:
Code:
a = "Python"
b = 100
print(a + b)
Output:
TypeError: can only concatenate str (not "int") to str
Now in example 1, we use a comma, which means we are printing both values so we get our values side by side in the result. But in example 2, we used the add sign, which means we are adding those values. But both variables have different data types. a is a string and b is an integer. And we cannot directly add an integer to a string. But we can add or concatenate the same data type. For example:
Example 3:
Code:
x = 100
y = 100
print(x, y)
Output:
100 100
Example 4:
Code:
x = 100
y = 100
print(x + y)
Output:
200
Now we can understand the difference when using print(x, y)
and print(x + y)
. When we use print(x, y)
, we are printing the values of x and y. But when we use print(x + y), we want the addition of those values. So this is the main difference.
Variables Naming Rules In Python
How to write the correct variable name is very important in Python. We can choose any name, except there are some rules that we should follow. These are the rules and suggestions that we should consider when writing variable names:
1: A variable name must start with a letter or the underscore (_) character. For example, age, name, _age, _name, first_name, car_name, etc. These are the valid names. We can start variable names with underscores and mix numbers too. For example, _data, _person, _person33, etc.
2: A variable cannot start with a number. We can use numbers with letters like data1, data33, name34age, age39, etc. These are valid names, but if we start with a number like 44data, 00age, etc. We will get SyntaxError. So we cannot start a variable name with a number.
3: A variable name can only contain alpha-numeric characters and underscores (A-Z, 0-9, and underscore).
4: Variable names are case-sensitive. Small and big letters are different in Python. For example: name, name, and NAME. Now these are three different variables in Python.
5: Variable name cannot have any reserved keywords. Reserved keywords are name, which is reserved for Python itself. For example, True is a reserve keyword in Python. So if we try to use True as a variable name, we will get SyntaxError.
6: Space or whitespace and any special characters or symbols are not allowed. (!, @, #,%, ^, *,& are not allowed). For example, first@name and first#name are both incorrect variable names.
So these are some rules for writing variable names. These rules are also called the Python variable naming convention.
How To Write Multiword Variable Name In Python
If we want to use a multiword name in a variable or a long name for a variable. For example:
Code:
allcomputersciencestudents = "Updated"
Here we are using multiword in variable name and because spaces are not allowed, it’s a little bit inconvenient to read. So there are some naming patterns that we can use:
Camel Case
In camel case, the first letter is in lowercase, and the first letter of other words is in uppercase.
Code:
allComputerScienceStudents = "Updated"
Explain:
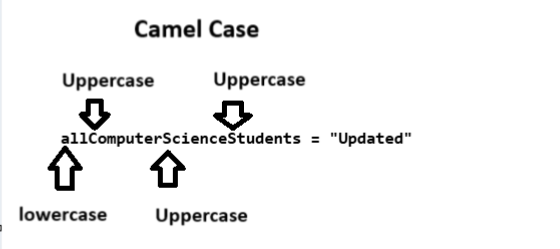
So in camel case, first letter in lowercase and first letter for other words in uppercase.
Pascal Case (CapWords)
In pascal case, the first letter of each word is in uppercase. This is also called CapWords style. For example:
Code:
AllComputerScienceStudents = "Updated"
Explain:
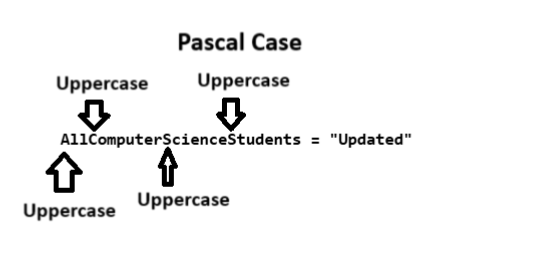
So the first letter of each word is in uppercase.
Snake Case
In snake case, we use a single underscore (_) character to separate the words. For example:
Code:
all_computer_science_students = "Updated"
Explain:
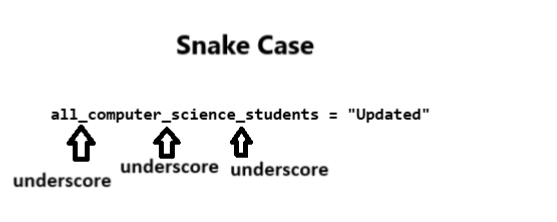
So in the snake case, we use an underscore between each word.
End of Python Variables Part 3
3 thoughts on “Python Variables (Part 3): Variables Output And Variables Naming Rules In Python”