Python type()
The type() is a built-in function in Python. We can use the type() function for two different purposes in Python. First, type() is used to find the data types of the object. This is the basic and mostly used purpose of type(). Second, we can create a new type object by using the type() function.
The syntax of Python type() is divided into two different categories. When we use type() to find out the data type, we use type() with a single argument. When we use type() to create a new type object, we use type() with three arguments.
Python type() with a single argument
We use type() with a single argument to find out the data type or class of the object.
Syntax: type(object) Object = Mandatory argument; we provide any kind of object, or values, or variable name. |
So we can find the class of any kind of object by using the type() function. For example:
Code:
data1 = 'This is Python programming.'
data2 = 104104
data3 = ['Python', 'Java', 2]
print(type(data1))
print(type(data2))
print(type(data3))
Output:
<class 'str'>
<class 'int'>
<class 'list'>
So we get the class or data type of the objects in the output. We pass the variables data1, data2, data3
as arguments in type parameters. If we want, we can directly use the values as arguments. For example:
Code:
print(type('This is Python.'))
print(type(100))
Output:
<class 'str'>
<class 'int'>
So in this example, we directly paste the values as arguments.
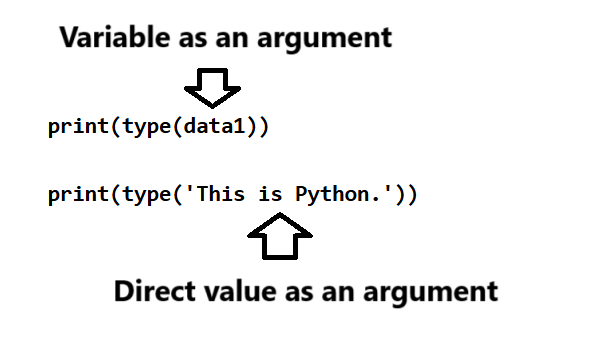
So whatever we pass between the parenthesis () is called an argument. This type() function takes only one argument. If we provide two arguments, then we will get an error.
Code:
print(type('This is Python.', 100))
Output:
TypeError: type() takes 1 or 3 arguments
We get an error. So either we provide only 1 argument or 3 arguments with the type() function but we provide 2 arguments.
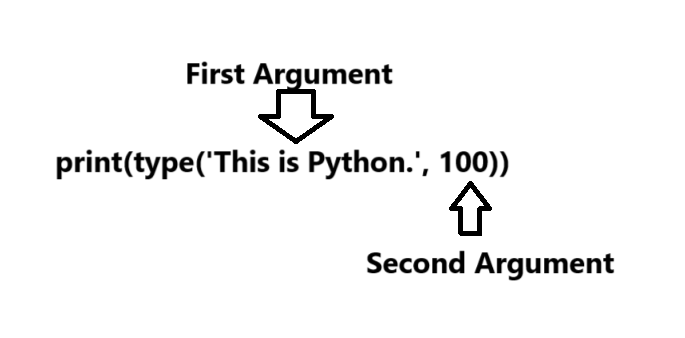
Now here we are trying to find out the class of both arguments. But this type() function takes only one argument so we get an error.
We can also use the type() function with a condition. For example:
Code:
print(type('Python') is str)
print(type('Python') is int)
Output:
True
False
In the upper example, we are checking the value 'Python'
is related to str class
or int class
. The output is shown in True and False. So instead of finding class by type() function, we can directly check if any value is related to a certain class or not. Now here is an important thing: we use 'str'
, not string. Same, we use 'int'
, not integer. So we have to provide the proper class name. For example:
Code:
print(type('Python') is string)
Output:
NameError: name 'string' is not defined.
So we get NameError, because we didn’t use the right class name.
We can also use type() with a conditional statement. For example:
Code:
x = 'This'
y = 'That'
if type(x) is type(y):
print('Yes, x and y has same data type.')
print('x and y has:', type(x))
else:
print('No, x and y has different data type.')
print('x has:', type(x))
print('y has:', type(y))
Output:
Yes, x and y has same data type.
x and y has: <class 'str'>
Here we use the type() function with an if and else statement. And we are checking if both variables (x, y) have the same data type or class. So because they both have the same string data type. So our if statment is correct and we get the print section of the if statement in the output. If we change the values, our output will change too. For example:
Code:
x = 'This'
y = 100
if type(x) is type(y):
print('Yes, x and y has same data type.')
print('x and y has:', type(x))
else:
print('No, x and y has different data type.')
print('x has:', type(x))
print('y has:', type(y))
Output:
No, x and y has different data type.
x has: <class 'str'>
y has: <class 'int'>
Now, because x is a string and y is an integer. So we get the else section in the output.
type() with expression or equation
We can also check an expression or equation with the type() function. Whatever the output of the expression is, decide the data type of that expression. For example:
Code:
print(type(11>2))
Output:
<class 'bool'>
So we get ‘bool’ in the output. Because the result of our expression (11>2) is True. And True represents the bool class so that’s why we get the ‘bool’ in the output. If we simply check an equation with type. For example:
type() with class object
We used classes to create objects in Python. We can use the type() function to find out the data type of the object. For example:
Code:
class Myclass:
print("We have created a new class")
Working = Myclass()
print(type(Working))
Output:
We have created a new class
<class '__main__.Myclass'>
We created an object with a class function and we use type() to find out the data type of this object.
So this is how we can use type() with single arguments.
type() with a statement
It is similar to how we learned in type() with an expression. Consider the following example:
Code:
first_list = ['Python', 'Java']
checking = 'Python' in first_list
print(type(checking))
Output:
<class 'bool'>
Here checking
is a variable that consists of a statment. And when we use type() with this variable, we get class 'bool'
in the output. Because the output of that statement is True (‘Python’ in first_list = True). And True is a boolean class data type so we get bool class in the output. So if we are finding the class of a statement, it is dependent on the output of that statement. Another example:
Code:
a = 1
b = 2
c = a + b
print(type(c))
Output:
<class 'int'>
Another example:
Code:
def first_function():
print('abc')
a = first_function.__doc__
print(type(a))
Output:
<class 'NoneType'>
We get ‘NoneType’ in the output because first_function.__doc__
means we are trying to find the docstring of this function but it doesn’t have any docstring. So a = None
is the output. And None is related to NoneType
.
Python type() with three arguments
We can use type() to create a new type of object. When we use type() with three arguments, then the type() function dynamically creates a new class and returns a new type of object.
Syntax: type(name, bases, dict) name: This is the first argument; we provide a class name. We can choose any name, but the name must be in strings. And this name is equal to __name__ attribute. bases: This is the second argument; in this argument, we provide the main base class name. And base class name must be in tuple. If there is no base class, then we write an empty tuple () or we can write (object). This base class name is equal to __bases__ attribute. dict: This is the third argument; in this argument we created a dictionary. This dictionary is helpful to creating the class. We can create a dictionary by both methods: dict(key = ‘Value’) or {‘key’ = ‘Value’}. This dictionary is equal to __dict__ attribute. |
Now we are going to create a new type object.
Code:
new = type('FirstClass', (), dict(a='Python'))
print(type(new))
Output:
<class 'type'>
Here we use type() as a constructor to create a new object. Here first argument = ‘FirstClass’, second argument = (), and third argument = dict(a=’Python’). We can also create this class like:
Code:
new = type('FirstClass', (object,), {'a': 'Python'})
print(type(new))
Output:
<class 'type'>
Here we changed the value of the second and third arguments. So this is how we can create a new type object
.
Python isinstance() Function
The Python isinstance() function is used to check whether the specified object is related to the specified class. If it is, then we get True in the output, and if it’s not, then we get False in the output. This function takes two arguments.
Syntax: isinstance(object, class) Object: First arguments; here we provide the object name or variable name or values. Class: Second arguments; here we write a class name. We can write more than one class name in a tuple. |
For example:
Code:
checking = isinstance(4, int)
print(checking)
Output:
True
Here we provide first argument = 4, second argument = int. Here we are checking that 4 is related to int class or not. And because 4 is an integer number, we get True in the output.
Code:
checking = isinstance(4, str)
print(checking)
Output:
False
We get False in the output because 4 is not related to the string class. In the second argument, we have to provide the class name, like str, int, bool, float, list, etc. If we use another name, we will get an error.
Code:
checking = isinstance(4, integer)
print(checking)
Output:
NameError: name 'integer' is not defined
So it is necessary to write the correct class name. We can write multiple class names in a tuple.
Code:
checking = isinstance(4, (int,str,float))
print(checking)
Output:
True
So here, we provide three class names in a tuple. And we are checking if 4 is related to one of them. And because it is. So we get True in the output. If the class is not found in a tuple, then the output will be False.
Code:
checking = isinstance(4, (list,str,float))
print(checking)
Output:
False
We can check sequence data type too. For example:
Code:
checking = isinstance(['Python', 'Java'], list)
print(checking)
Output:
True
So here we are checking list data type. We can provide arguments as variables too. For example:
Code:
a = (1,2,3)
b = tuple
print(isinstance(a,b))
Output:
True
If we provide more than one object or more than one class without using tuples, then we will get an error. For example:
Code:
checking = isinstance('python', 100, str, int)
print(checking)
Output:
TypeError: isinstance expected 2 arguments, got 4
So we get a TypeError, and it’s clearly showing that the instance() function required only 2 arguments.
We can also check a class object with the isinstance() function. For example:
Code:
class MyClass:
value = "Python"
first_object = MyClass()
print(isinstance(first_object, MyClass))
Output:
True
Here we created an object and checked it with the isinstance() function.
Difference Between type() and isinstance() Function
There are some differences between the type() and isinstance() functions.
Syntax: Both functions have different syntax. isinstance() takes 2 arguments, while type() takes either 1 or 3 arguments. |
Purpose: isinstance() is used when we know the class but want to make sure of it. While type() is used to find out the class and also used to create a new type object. |
inheritance: Through the isinstance() we can find the class and subclass for an object. But we cannot do such thing with type(). |
Faster: isinstance() is faster in comparison to type(). |
Multiple Classes: In isinstance(), we can provide multiple classes as arguments, while we cannot do such thing with the type() function. |
Conclusion
This is the end of the Python type() tutorial. We learned about type() and isinstance() function. Both are related to classes. type() is used when we want to find out the class or data type of an object. And isinstance() is used when we know the class name but want to make sure of it.
End of the Python type() tutorial.
2 thoughts on “Python type(): What are type() and isinstance() functions in Python?”