Python input()
In this tutorial, we will learn about the Python input() function. So what is the input() function in Python? The input() function in Python is a way to interact with the user. We can take input from the user by using the input() function. Suppose we want some information from the user, like name, age, place, etc., then we use the input() function. We create a program with the help of the input() function and this program will not stop until the user provides the input.
The input() function is a built-in function in Python. We don’t need to import any module to use the input() function. In Python 2, we used raw_input() function instead of input() function. In Python 3, we use input() instead of raw_input(). Syntax for input() function:
Syntax: input(‘Prompt) Prompt (optional): Only one argument is required and it is also optional. We write a string in prompt, which we want to show the user while taking the input. Most important thing, whatever the user writes, the input() function always returns a string. |
How to use the input() function in Python?
There are two ways to use the input() function in Python.
1: Without Prompt |
2: With Prompt |
Python input() function without prompt
We can use the input() function without the prompt argument. For example:
Code:
print("Enter Your Name: ")
name = input()
print('Hello!', name)
Output:
Enter Your Name:
Now when we run this code. We get this line in the output, Enter Your Name: The execution of the program will stop until the user enters a name. So the interpreter waits for the user to provide any input. After that, the user writes their name and presses enter. Pressing enter is necessary. And then the last print line will be executed. For example, if the user writes Python as a name.
Code:
print("Enter Your Name: ")
name = input()
print('Hello!', name)
Output:
Enter Your Name:
Python
Hello! Python
So we get Hello! Python
in the output. So this is how we can take input from the user. Now back to understanding the code line. In the first line, we write, print(“Enter Your Name: “) This is like the instruction for the user. And also, this is necessary because we are using the input() function without prompt. So after the first line, we create a variable name and here we set name = input()
so whatever the user input will be, it will hold into the name variable. In the third line, we write, print('Hello!', name)
This is the message we want to print after the user enters a name. For better understanding:
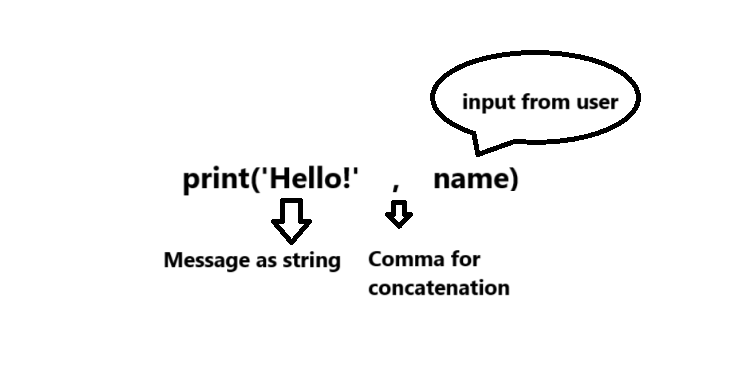
So we write ‘Hello!’ as a message; we can write anything instead of ‘Hello!’, then we concatenate this message with the user input. So user input was Python. So we get Hello! Python
in the output.
Another example for better understanding:
Code:
print("Enter Your Name: ")
name = input()
print('Hello!', name)
print('Where are you from?')
place = input()
print('Hello', name, 'from', place)
Output:
Enter Your Name:
Python
Hello! Python
Where are you from?
all around the world
Hello Python from all around the world
Here we make a simple program. We add another input() in this program. After the Hello! Python
Our program asks the location from the user. And the user enters their location (all around the world) and in the last line, we add both inputs with the string messages.
In the upper example, we use a comma to concatenate the string and user input. We can also use add (+) sign instead of comma. However, both have different meanings. Click here to learn the difference between a comma and an add sign while printing.
So if we want, we can use the add sign instead of comma and we can also use f-string. For example:
Code:
print("Enter Your Name: ")
name = input()
print('Hello ' + name)
Output:
Enter Your Name:
Python
Hello Python
We get the same output. We can also use f-string.
Code:
print("Enter Your Name: ")
name = input()
print(f'Hello {name}. Good day.')
Output:
Enter Your Name:
Python
Hello Python. Good day.
So these are the different options we can choose according to our uses. We already learned that the input() function always returns a string data type. We can check this by using the type() function.
Code:
print("Enter Your Name: ")
name = input()
print('Hello ' + name)
print(type(name))
Output:
Enter Your Name:
Python
Hello Python
<class 'str'>
So we get the ‘str’ class in the output. Don’t get confused that users write their name in alphabet so we get the ‘str’ class in the output. It doesn’t matter what the user enters; the input() function always returns the ‘str’ class. For example:
Code:
print("Enter Your Name: ")
name = input()
print('Hello ' + name)
print(type(name))
Output:
Enter Your Name:
104
Hello 104
<class 'str'>
Now users enter a number instead of a name, but we still get the ‘str’ class in the output.
If we are concatenating user input with an integer data type, then we have to use the type casting. For example:
Code:
print("Enter Your birth Year: ")
number = input()
current_year = 2025 - number
print(f"You are {current_year} old.")
Output:
Enter Your birth Year:
1991
TypeError: unsupported operand type(s) for -: 'int' and 'str'
We get an error in the output because we already learned that the input() function always returns a string data type. Now in the second line, when the user writes their year of birth (1991),. However, the user writes a number but still it is a string. Now in the third line of code, we are trying to subtract the user input from the year 2025. It means we are trying to subtract 1991 from 2025. Which is right but 1991 is a string and 2025 is an integer data type. We cannot do direct operations between different data types. That was the error indicated: unsupported operand for -: ‘int’ and ‘str’.
To solve this problem, we have to use casting. We convert the string data type into the integer data type. For this, we use the int() function.
Code:
print("Enter Your birth Year: ")
number = input()
current_year = 2025 - int(number)
print(f"You are {current_year} old.")
Output:
Enter Your birth Year:
1991
You are 34 old.
Here we used casting; in the third line we changed the user input into the integer data type.
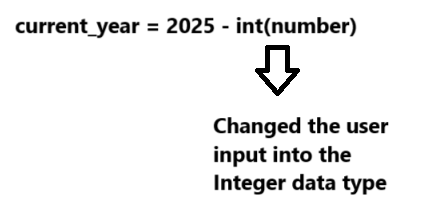
So we get the correct output.
Now if the user enters any other input instead of a number,. For example:
Code:
print("Enter Your birth Year: ")
number = input()
current_year = 2025 - int(number)
print(f"You are {current_year} old.")
Output:
Enter Your birth Year:
Python
ValueError: invalid literal for int() with base 10: 'Python'
Here the user enters Python
as input but now our program can only accept numbers. So we get an error.
Python input() function with prompt
In the upper section, we learned about the input() function without prompt. We can use the input() function with prompt. Now, because we are using prompt arguments, we don’t have to use the print function for messages. For example:
Code:
name = input('Enter your name: ')
print('Hello!', name)
Output:
Enter your name: Python
Hello! Python
So this is how we can use prompt arguments with an input function. In this example, the user writes their name in the same line. If we want, we can change this and allow the user to enter their name in a new line. For example:
Code:
name = input('Enter your name: \n')
print('Hello!', name)
Output:
Enter your name:
Python
Hello! Python
Here we use an escape character for a new line.
Following is an example of multiple inputs from the user:
Code:
name = input('What is your name?: ')
age = input('How old are you?: ')
fav_color = input('What is your favorite color?: ')
print(f'Hello {name}. You are {age} years old and your favorite color is {fav_color}.')
Output:
What is your name?: V
How old are you?: 33
What is your favorite color?: Red
Hello V. You are 33 years old and your favorite color is Red.
So this is how we can take multiple inputs from the user.
Conclusion
This is the end of the Python input() tutorial. input() function is very important in Python. Because it allows the programmer to interact with the user.
End of the Python input() tutorial.
One thought on “Python input(): What is the input() function in Python?”