Click For Python Variables Part 3
Types Of Variables in Python
There are two types of variables: global and local. When we are working with functions, then we have to use global and local variables. So we have to understand the difference and working of local and global variables.
Global Variables In Python
Normally, when we create a variable outside the function, by default, it is a global variable. Global variables can be used by everyone. It can be accessed anywhere in the whole program.
Normally we cannot create a global variable inside the function. Global variables are created outside of a function. However, it can be accessed inside or outside the function. By default, a variable declared outside of the function is a global variable.
Code:
x = "Python"
y = "Java"
def first_function():
print(x,y)
print(x,y)
first_function()
Output:
Python Java
Python Java
Explanation of Code:
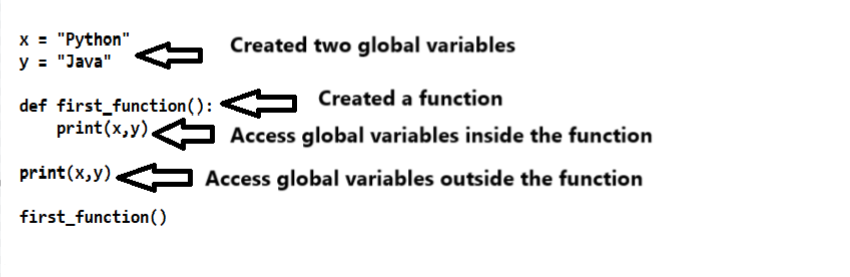
Here we created two variables x and y; by default, both variables are global variables because they are not under any function. Basically, they are free so anybody can access them. After that, we created a function named first_function()
Under this function, we are using print(x, y).
We are trying to print the value of x and y. And because x and y are global variables. So we can access them anywhere in our program.
After that, we used another print(x, y)
this print function is outside the function. Then we simply call the function in the last line. So we created two global variables and tried to access them from inside the function and outside the function.
So we get the output; both print functions return the variable values.
So in basic terms, we can say that if we create a variable in a normal way, it is a global variable.
Local Variables In Python
Local variables are created inside the function and they can be accessed only inside the function. They cannot be accessed anywhere in the program. A local variable created inside a function can only be accessed inside that function. If we try to access them outside the function, we will get an error. For example:
Code:
first_global = "Python"
def first_function ():
first_local = "Java"
print(first_local)
print(first_global)
first_function()
Output:
Python
Java
Explanation of Code:
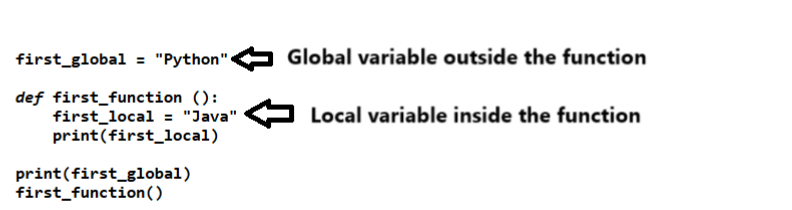
Here we created a global variable outside the function named first_global
, then we created a function and inside this function we created a local variable. We don’t have to specify the type of variable. By default, a variable outside the function is treated as global and a variable inside the function is treated as local.
We simply used print(first_global)
, which means we are trying to access a global variable outside the function and it is possible. Now what if we try to access the local variable outside the function? For example:
Code:
first_global = "Python"
def first_function ():
first_local = "Java"
print(first_local)
print(first_local)
Output:
NameError: name 'first_local' is not defined.
We get NameError because we are trying to access the local variable outside the function. So first_local
is a local variable and we can access this variable inside the function, not outside the function. But as we learn in global variables, global variables can be accessed anywhere in the program. For example:
Code:
first_global = "Python"
def first_function ():
first_local = "Java"
print(first_local)
print(first_global)
first_function()
Output:
Java
Python
Under the function, we use print(first_local)
and print(first_global)
; we are trying to access global and local variables inside the function. So global variables can be accessed anywhere in the program.
So this is the difference between local and global variables.
Local And Global Variables With Same Variable Name
In the following example, we will learn how our program will run if we create the same named variable inside and outside the function.
Code:
a = "Python"
b = "Java"
def first_function():
a = "Not Python"
b = "Not Java"
print(a,b)
print(a,b)
first_function()
Output:
Python Java
Not Python Not Java
We created the same named variable (a, b)
inside and outside the function. Then we access both variables outside and inside the function.
We get the first result from the global variable because we are accessing the global variable. And we get the second result from the local variable because we call the function and the function prints the value of the local variable. Now here is an important thing: Why the function didn’t use the global variable? because whenever we call the function, first it will search for the variable inside the function, and if it isn’t found, then it will search and use the global variable. But here we already created the variable inside the function. So it won’t need to use the global variable. Now if we change the situation:
Code:
a = "Python"
b = "Java"
def first_function():
a = "Not Python"
print(a,b)
print(a,b)
first_function()
Output:
Python Java
Not Python Java
Now in the second result, the first value is Not Python
means, a = Not Python
and b = Java
. Function didn’t have b, so it accessed the global variable b and printed it in the output. So every function first will look within for a variable; if not found, then use the global variable.
Note: We used the same variable name just for learning purposes; it is not recommended to use the same name for global and local variables unless it is required.
Difference Between Identifier And Variable In Python
Identifier and variable are related but not exactly the same thing. An identifier is a name given to entities like classes, functions, variables, modules, etc. So a variable is only a kind of identifier, just like other kinds of identifiers are function names, class names, structure names, etc. So we can say all variables are identifiers.
So when we provide a name for the identifier,. The rules are the same as we learn in variable naming rules. Like in our example, when we created a function, we provided a name for the function; we called it first_function
. The rules we applied to write a variable name are also applied when writing a function name. So now this function name is an identifier.
In simple words, we can say, “An identifier is a name given to entities in Python, while a variable is a specific type of entity that holds a value in memory and is referenced by an identifier.”
See the below example for more clarity:
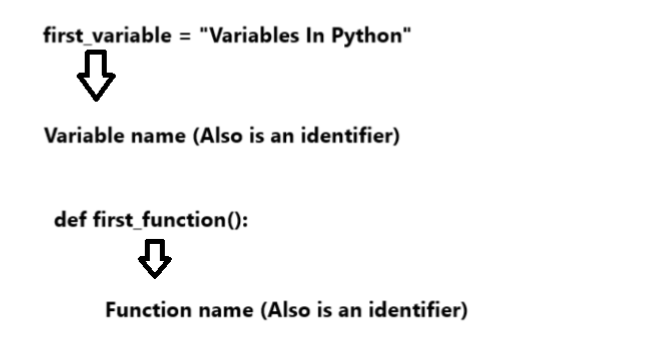
Delete a Variable In Python
When we don’t need a variable. It’s good to delete that variable. So it could free space and also free the name it holds.
To delete a variable. We use del keyword.
Code:
a = "Python"
print(a)
del a
print(a)
Output:
Python
NameError: name 'a' is not defined
To delete a variable. We simply write del and then provide the variable name.
Conclusion
This is the end of the Python variable tutorial. Variables are very important in Python because if you want to make a short program or a big program, you will have to use variables. Variables is a basic concept in Python. So always follow the rules when creating variables. Always remember Python is a case-sensitive language.
End of Python Variables Part 4
2 thoughts on “Python Variables (Part 4): What are Local and Global Variables? Identifier vs. variables?”